Here was my submission for my 4th yr engineering project. Its a multi-input interface that studies the applications of a touch-less interface in user-PC interactivity. Keep in mind this interface does not have to be limited to PCs itself and could also be used in interaction with other electronics such as TVs etc.
Enjoy!
Saturday, August 09, 2008
Tuesday, August 05, 2008
Trying to run your DirectX or other VC++2008 app on another computer ? Heres what you need....
I was trying out some of my Wii project code on another computer today. Its a directX app that uses the Wiimote input for interaction and i kept getting the errors
This application has failed to start because the application configuration is incorrect. Reinstalling the application may fix this problem.
Heres the sollution:
This application has failed to start because the application configuration is incorrect. Reinstalling the application may fix this problem.
Heres the sollution:
- rebuld the application in release form and not debug form. in visual C++ 2008 go to Build > Configuration manager and in the "Active sollution configuration" select "Release". Now rebuild the app. You may several errors, they are covered earlier int the blog.
- Heres the important part. A VC++2008 app requires certain libraries to run on another computer and if that computer doesnt have visual studio you get the Microsoft Visual C++ 2008 redistributable package and this will provide your app the proper envirnment to run in.
Labels:
directx,
microsoft,
redistributable,
visual c++ 2008,
visual studio
Saturday, July 19, 2008
ever get the frusterating error:
error C2664: 'CWnd::MessageBoxW' : cannot convert parameter 1 from 'const char [12]' to 'LPCTSTR'
Heres a sollution:
Change your project configuration to use multibyte strings. Press ALT+F7 to open the properties, and navigate to Configuration Properties > General. Switch Character Set to "Use Multi-Byte Character Set".
Another issue when doing 3d programming is when the libraries in Visual Studio do no import the necessary functions and you get Link errors. heres the sollution:
Change your project configuration to use multibyte strings. Press ALT+F7 to open the properties, and navigate to Configuration Properties > Linker > Input. The option Additional dependencies should read "$(NOINHERIT)". click it and select the "..." button that appears beside it.This shows all the dependencies available. Make sure the checkbox "Inherit from parent or project defaults" is checked. The code should now compile.
error C2664: 'CWnd::MessageBoxW' : cannot convert parameter 1 from 'const char [12]' to 'LPCTSTR'
Heres a sollution:
Change your project configuration to use multibyte strings. Press ALT+F7 to open the properties, and navigate to Configuration Properties > General. Switch Character Set to "Use Multi-Byte Character Set".
Another issue when doing 3d programming is when the libraries in Visual Studio do no import the necessary functions and you get Link errors. heres the sollution:
Change your project configuration to use multibyte strings. Press ALT+F7 to open the properties, and navigate to Configuration Properties > Linker > Input. The option Additional dependencies should read "$(NOINHERIT)". click it and select the "..." button that appears beside it.This shows all the dependencies available. Make sure the checkbox "Inherit from parent or project defaults" is checked. The code should now compile.
Tuesday, June 24, 2008
Opening a serial port in C#
Just a quick tutorial, I was trying to communicate with my LCD in C# and heres the gist of how i got it up and running:
Use the Header using System.IO.Ports; we create an object using the name serial_port which looks like:
Use the Header using System.IO.Ports; we create an object using the name serial_port which looks like:
SerialPort serial_port = new SerialPort("COM7", 9600, Parity.None, 8, StopBits.One);
Here we specify the required vars - Com port name, Baud rate, Parity bit, Data bits and stop bits. Now we simply open the com port serial_port.Open(); and this allows us to read/write from the selected port. For my purposes I used write commands so as to write data to my external LCD screen. Keep in mind the write command accepts only strings so I had to improvise as I was sending it a integer value.
serial_port.Write( i.ToString() );
where 'i' is the integer I am sending accross the COM7 port.
Sunday, June 08, 2008
Establishing a basic connection with the Wiimote
Been working on coding for the Wiimote using C# and have thankfully made some progress. Import the wiimote library and create an object for the wiimote
Now in the main function
This establishes the connection with the wiimote and the SetLeds tells the wiimote to show the first of four lights on the controller.
This saves the coordinates in our strings x and y. You can take a printout or do whatever you want with these values now, still not sure what rate the wiimote is streaming the data at though.
using WiimoteLib;
Now in the main function
wm.Connect();
wm.SetReportType(InputReport.IRAccel, true);
wm.SetLEDs(true, false, false, false);
wm.SetReportType(InputReport.IRAccel, true);
wm.SetLEDs(true, false, false, false);
This establishes the connection with the wiimote and the SetLeds tells the wiimote to show the first of four lights on the controller.
x = wm.WiimoteState.IRState.IRSensors[0].Position.X.ToString();
y = wm.WiimoteState.IRState.IRSensors[0].Position.Y.ToString();
y = wm.WiimoteState.IRState.IRSensors[0].Position.Y.ToString();
This saves the coordinates in our strings x and y. You can take a printout or do whatever you want with these values now, still not sure what rate the wiimote is streaming the data at though.
Sunday, May 04, 2008
Capstone Project: Headtracking using Wiimote
I am creating a project similar to Johny Lee's headtracking project using the Nintendo Wii remote for my final year engineering design project. 1st step is ofcourse to get the remote up and running with the existing applications created by Mr Lee. The following steps are required:
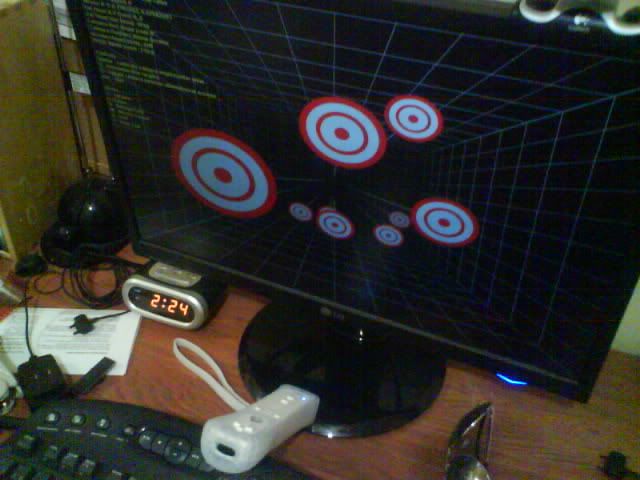
The next few posts will include a dissection of the code in order to fully understand what is going on here.
- Establish a bluetooth connection with the wiimote. Use the tutorial here to accomplish that. After you sync the Wiimote the lights on the remote will continue flashing as if its in sync mode, this is normal as the remote only syncs to controller 1 after the application interfaces with it.
- Download and install the latest .NET version and the DirectX SDK for the application to run properly. I spent a lot of time figuring out why the application kept crashing upon startup and it turned out i needed the DirectX SDK and not just DirectX 9.0c .
- Fire up the WiiDesktopVR.exe
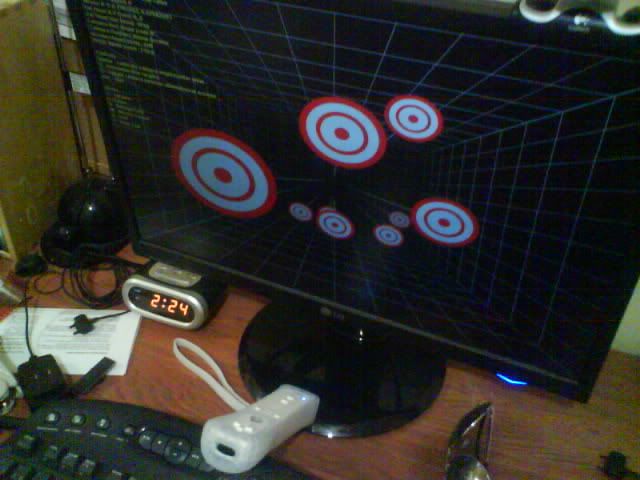
The next few posts will include a dissection of the code in order to fully understand what is going on here.
Labels:
capstone,
engineering,
johhny lee,
tracking,
wii,
wiimote
Subscribe to:
Posts (Atom)